\ |
_ \ __ \ _` | __ \ | / _ \
___ \ | | ( | | | < __/
_/ _\ _| _| \__,_| _| _| _|\_\ \___|
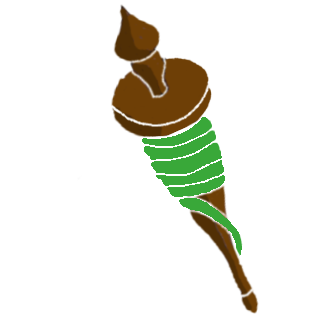
The programming language
"Should I care?"
RESULT: Please fill in the form
Note: Ananke is currently not implemented. Work is ongoing to build a reference compiler. check out the repo.
For syntax examples, check back soon.
There is a WIP editor: the editor
Philosophy
Ananke is a compiled application programming language that throws away a lot of conventions. The pay off is deep consistency and composability. There's a focus on static analysis and correctness, balanced with flexibility and expressivity through duck typing and an expressive grammar.
Syntax:
Ergonomic to read and write
Grammar:
Simple and compact
Features:
Orthogonal concerns handled orthogonally
Semantics:
Consistent
Typesystem:
Intelligent and flexible, constraining only where relevant
Experience:
Things just work: the compiler will figure it out
Language:
Powerful and free of dogma
You should be given all the power. It is up to you, the chef, to not turn delicious, exotic, and rare ingredients into demonic spaghetti.
If you want to use the best tool for the job, what good is a toolbox that doesn't have it?
Notable Features
- Unlimited precision integer and rational numbers, by default
- At last, you can perform currency calculations without a library!
- Monadic error handling
- Optional: either something, or
nil
- By default, nothing is
nil
, even references
- By default, nothing is
- Error: either something, or an error
- Syntax sugar for unpacking and handling the edge case
- Optional: either something, or
- Untedious syntax
- Common operations are short operations
- Nested long comments
- SIMD: just use vectors
- Vector math like
[1,2,3] + [4,5,6]
- Ruby-like loop controls
- Named loops and breaking out of nested loops
- Undelimited continuations
- Coroutines
- Generators and iterators
- Exceptions
- Resumable exceptions
- "Effect handlers"
- "Lisp Conditions"
- Pattern matching
- Destructuring assigment
- Match expressions (
case
) - You can define functions piecewise
- Value semantics
- Function arguments are passed by copy (value)
- Data is stored inline, better for CPU cache
- No "const ArrayList, but mutable elements" malarkey
- "R-values" are just values
- "L-values" are just references to values
- "Pointers" are just references to values
- Pre-emptive multithreading
actor
s that cansend
andreceive
messages- Synchronize code and data with the
atom
modifier
- Automatic memory management
- Novel reference counting algorithm that deals with cycles
- Everything is an expression
- Uniform function call syntax
f(x)
is the same asx.f()
- Method chaining? Pipes? It has never been this easy!
- Strong static duck typing
- Types can be inferred
- Contracts and refinements (such as ranges) with
where
- Generics: polymorphic functions and data structures
- Algebraic types: variants and records
- Also their anonymous counterpart, enums and tuples
- Interfaces can be implicit, yet have a stable ABI
- Static unit system
- Dimensional analysis
- Automatic conversions and inference
- "point" and "difference" units: temperature differences won't wrongly convert.
- Precise control over data layout
exact
modifier for bit-perfect record layouts- Modifiers for endianness
- Data types whose bit size can be specified
- First class functions
- Higher order functions
- Nested functions
- Closures with access to parent variables
- Recursion, with tail call elimination
- Currying and un-currying affordances
- Metaprogramming
- Reflections
- Compile time evaluation
symbol
data type- Syntax tree macros
Why not write Ananke?
- Obscure language
- No support for inheritance
- Not a lot of libraries available
- In fact, no working implementation exists
- In super fact, the language specification is still under construction
- Your boss will likely shoot you if you suggest using it in prod at this point